In the dynamic world of mobile app development, React Native has become a leading framework for building cross-platform applications. A common requirement for developers is to incorporate an expand icon React Native to enhance user interaction. This feature is frequently used in components like dropdowns, accordions, and expandable lists. In this article, we will delve into everything you need to know about implementing an expand icon in React Native, from its importance to practical coding examples.
What is an Expand Icon React Native?
An expand icon React Native is a visual indicator that allows users to toggle between expanded and collapsed views. It is often represented by a chevron, arrow, or plus symbol, which changes its orientation or appearance depending on the current state. This functionality is integral to user-friendly interfaces, providing a way to reveal or hide additional content.
For example, an FAQ section in an app may use an expand icon to allow users to view answers by expanding questions. Similarly, dropdown menus or expandable cards leverage this feature to maintain a clean design while giving users access to more information.
Also Read: Ztec100.com
Why Use an Expand Icon React Native?
The expand icon React Native serves several purposes:
- Improved User Experience: It keeps the interface clean and organized by hiding unnecessary details until needed.
- Space Optimization: Expandable elements allow you to present extensive information in limited screen space.
- Enhanced Navigation: With expand icons, users can navigate through hierarchical content intuitively.
- Interactivity: Adding interactivity boosts engagement, as users can actively control what they want to see.
React Native makes it easy to implement this feature, offering various tools, libraries, and components that can be customized to meet your app’s design needs.
How to Implement an Expand Icon React Native
To integrate an expand icon React Native, you can follow different approaches. Below, we’ll explore some common methods, from using popular libraries to creating custom implementations.
Using React Native Vector Icons
One of the most popular ways to add an expand icon is by using the react-native-vector-icons library. This library provides a vast collection of icons, including chevrons and arrows, which are ideal for expand functionality.
Installation:
To begin, install the library using the following command:
npm install react-native-vector-icons
Example Code:
Here’s how you can use an expand icon in an accordion component:
import React, { useState } from ‘react’;
import { View, Text, TouchableOpacity } from ‘react-native’;
import Icon from ‘react-native-vector-icons/Ionicons’;
const ExpandableSection = () => {
const [expanded, setExpanded] = useState(false);
return (
<View>
<TouchableOpacity
onPress={() => setExpanded(!expanded)}
style={{ flexDirection: ‘row’, alignItems: ‘center’ }}
>
<Text style={{ marginRight: 10 }}>{expanded ? ‘Collapse’ : ‘Expand’}</Text>
<Icon name={expanded ? ‘chevron-up’ : ‘chevron-down’} size={24} color=”black” />
</TouchableOpacity>
{expanded && (
<View style={{ marginTop: 10 }}>
<Text>This is the expanded content!</Text>
</View>
)}
</View>
);
};
export default ExpandableSection;
In this example, the chevron-up and chevron-down icons indicate the expanded and collapsed states respectively.
Creating Custom Expand Icons
If you prefer not to use external libraries, you can create your own expand icon in React Native using SVG or even plain text. The react-native-svg library is a powerful tool for creating scalable and customizable icons.
Installation:
Install the library with this command:
npm install react-native-svg
Example Code:
import React, { useState } from ‘react’;
import { View, Text, TouchableOpacity } from ‘react-native’;
import { Svg, Path } from ‘react-native-svg’;
const ExpandableSection = () => {
const [expanded, setExpanded] = useState(false);
return (
<View>
<TouchableOpacity
onPress={() => setExpanded(!expanded)}
style={{ flexDirection: ‘row’, alignItems: ‘center’ }}
>
<Text style={{ marginRight: 10 }}>{expanded ? ‘Collapse’ : ‘Expand’}</Text>
<Svg width=”24″ height=”24″ viewBox=”0 0 24 24″>
<Path
d={expanded ? ‘M6 15l6-6 6 6’ : ‘M6 9l6 6 6-6’}
fill=”none”
stroke=”black”
strokeWidth=”2″
/>
</Svg>
</TouchableOpacity>
{expanded && (
<View style={{ marginTop: 10 }}>
<Text>This is the expanded content!</Text>
</View>
)}
</View>
);
};
export default ExpandableSection;
In this code, the expand icon is dynamically generated using SVG paths, making it lightweight and fully customizable.
Also Read: Money6x.com
Comparing Libraries and Custom Implementations
To help you decide the best method, here’s a comparison of the two approaches:
Feature | Libraries (e.g., Vector Icons) | Custom Implementation |
Ease of Use | Very easy to integrate | Requires coding effort |
Customization | Limited to library features | Fully customizable |
Performance | Slightly heavier | Lightweight |
Scalability | Good for standard use cases | Best for unique needs |
Best Practices for Using Expand Icon React Native
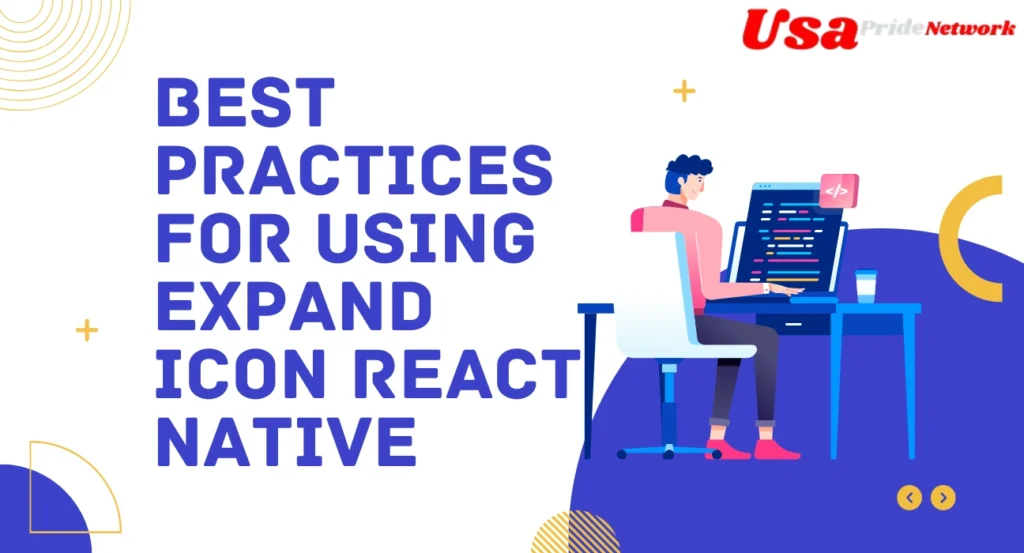
When using an expand icon React Native, there are some best practices to follow:
- Keep It Intuitive: The icon should clearly indicate its function. Use familiar symbols like arrows or plus signs.
- Ensure Accessibility: Add accessibility labels to make the icons usable for screen readers.
- Smooth Transitions: Use animations to make the expansion and collapse visually appealing.
- Test Responsiveness: Ensure the icon and content behave correctly across different screen sizes.
Enhancing User Interaction with Animations
To improve the user experience, consider adding animations to your expand icon in React Native. The Animated API allows you to create smooth transitions between states. For example, you can rotate an arrow icon to indicate the toggle action.
Here’s an example of a rotating icon:
import React, { useState, useRef } from ‘react’;
import { View, Text, TouchableOpacity, Animated } from ‘react-native’;
const AnimatedExpandableSection = () => {
const [expanded, setExpanded] = useState(false);
const rotation = useRef(new Animated.Value(0)).current;
const toggleExpand = () => {
Animated.timing(rotation, {
toValue: expanded ? 0 : 1,
duration: 300,
useNativeDriver: true,
}).start();
setExpanded(!expanded);
};
const rotate = rotation.interpolate({
inputRange: [0, 1],
outputRange: [‘0deg’, ‘180deg’],
});
return (
<View>
<TouchableOpacity onPress={toggleExpand} style={{ flexDirection: ‘row’, alignItems: ‘center’ }}>
<Text style={{ marginRight: 10 }}>{expanded ? ‘Collapse’ : ‘Expand’}</Text>
<Animated.View style={{ transform: [{ rotate }] }}>
<Text>▼</Text>
</Animated.View>
</TouchableOpacity>
{expanded && (
<View style={{ marginTop: 10 }}>
<Text>This is the expanded content!</Text>
</View>
)}
</View>
);
};
export default AnimatedExpandableSection;
Frequently Asked Questions
How do you implement an expand icon React Native?
To implement an expand icon in React Native, you can use libraries like react-native-vector-icons or create custom SVG icons using the react-native-svg library. These tools make it easy to toggle between expanded and collapsed states.
What is the best library for expand icons in React Native?
The react-native-vector-icons library is the most popular choice for expand icons. It offers a wide range of prebuilt icons, such as chevrons and arrows, that can be easily customized and integrated.
Can I create a custom expand icon in React Native?
Yes, you can create a custom expand icon using react-native-svg for scalable vector graphics or by styling text-based symbols (like ▲ or ▼) for a lightweight solution.
How can I add animation to an expand icon in React Native?
Use the Animated API in React Native to rotate or transform the expand icon during state changes. This enhances the user experience with smooth visual transitions.
Conclusion
The expand icon React Native is an essential feature for modern app interfaces, enabling developers to present information efficiently and interactively. Whether you opt for libraries like react-native-vector-icons or prefer custom implementations, the flexibility of React Native makes it easy to adapt this feature to your specific needs. By following best practices and incorporating animations, you can ensure a seamless and engaging user experience. As React Native continues to evolve, mastering components like expand icons will remain crucial for creating dynamic and responsive applications.